字符串
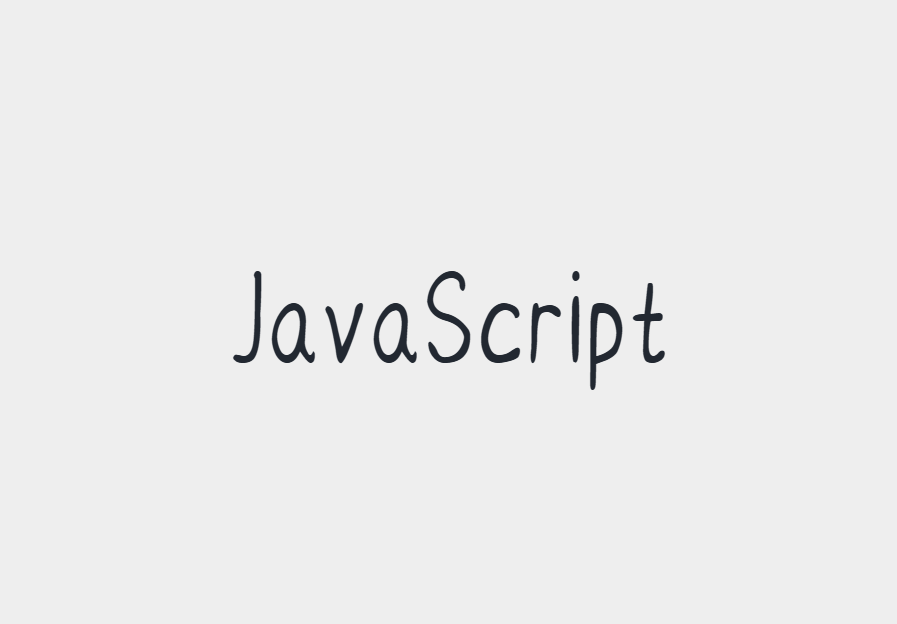
字符串
寻觅~流光字符串
一、字符串的基本定义与特性
1. 定义方式
- 双引号:
"hello"
- 单引号:
'hello'
- 反引号(模板字符串):
hello
2. 字符串的不可变性
字符串是不可变类型,一旦创建就无法更改其内容,只能生成新的字符串。
1
2
3let str = "hello";
str[0] = "H"; // 无效
console.log(str); // "hello"
3. 字符串与 Unicode
JavaScript 使用 Unicode 编码,每个字符占用 2 个字节。
可以使用转义字符表示特殊字符,例如:
1
let str = "Hello\u0041"; // "HelloA"
二、字符串的常用操作
1. 字符串的长度
使用 .length属性获取字符串的长度:
1
2let str = "hello";
console.log(str.length); // 5
2. 字符串访问
使用索引访问字符串中的字符:
1
2let str = "hello";
console.log(str[1]); // "e"
三、字符串方法
1. 字符串的提取方法
**
slice(start, end)
**:提取指定范围的子字符串(不包括end
)。1
2let str = "hello world";
console.log(str.slice(0, 5)); // "hello"**
substring(start, end)
**:类似slice
,但不支持负数索引。1
console.log(str.substring(0, 5)); // "hello"
**
substr(start, length)
**:从指定位置提取指定长度的子字符串(已废弃)。1
console.log(str.substr(0, 5)); // "hello"
2. 字符串的查找方法
**
indexOf(substring, start)
**:返回子字符串的首次出现索引,没有则返回-1
。1
console.log(str.indexOf("o")); // 4
**
lastIndexOf(substring)
**:返回子字符串的最后一次出现索引。1
console.log(str.lastIndexOf("o")); // 7
**
includes(substring)
**:检查字符串是否包含子字符串。1
console.log(str.includes("world")); // true
**
startsWith(substring)
**:检查字符串是否以子字符串开头。1
console.log(str.startsWith("hello")); // true
**
endsWith(substring)
**:检查字符串是否以子字符串结尾。1
console.log(str.endsWith("world")); // true
3. 字符串的修改方法
toUpperCase()
和 **toLowerCase()
**:将字符串转换为大写或小写。1
2let str = "Hello";
console.log(str.toUpperCase()); // "HELLO"**
trim()
**:去除字符串首尾的空格。1
2let str = " hello ";
console.log(str.trim()); // "hello"**
replace(search, replacement)
**:替换匹配的子字符串。1
console.log(str.replace("hello", "hi")); // "hi"
**
replaceAll(search, replacement)
**:替换所有匹配的子字符串。1
2let str = "hello hello";
console.log(str.replaceAll("hello", "hi")); // "hi hi"
4. 字符串的分割和连接
**
split(separator)
**:将字符串按照指定分隔符分割为数组。1
2let str = "a,b,c";
console.log(str.split(",")); // ["a", "b", "c"]**
concat(...strings)
**:连接多个字符串。1
2
3let str1 = "hello";
let str2 = "world";
console.log(str1.concat(" ", str2)); // "hello world"
5. 字符串的填充
**
padStart(targetLength, padString)
**:在字符串开头填充。1
console.log("5".padStart(3, "0")); // "005"
**
padEnd(targetLength, padString)
**:在字符串末尾填充。1
console.log("5".padEnd(3, "0")); // "500"
6. 字符串的比较
字符串可以通过 **>、<**、**>=**等运算符进行字典序比较。
1
console.log("apple" > "banana"); // false
7. 字符串的重复
repeat(n)
:重复字符串 n次。1
console.log("hello".repeat(3)); // "hellohellohello"
四、模板字符串(Template Literals)
1. 基本语法
使用反引号定义,支持多行字符串和嵌入变量。
1
2let name = "Alice";
console.log(`Hello, ${name}!`); // "Hello, Alice!"
2. 表达式嵌入
模板字符串可以嵌入任意表达式。
1
console.log(`2 + 2 = ${2 + 2}`); // "2 + 2 = 4"
3. 标签模板
使用函数对模板字符串进行处理。
1
2
3
4
5function tag(strings, ...values) {
console.log(strings); // 模板字符串的静态部分
console.log(values); // 嵌入的表达式
}
tag`Hello, ${name}!`;
五、字符串与其他类型的转换
1. 转为字符串
使用
String()
函数或.toString()
方法。1
console.log(String(123)); // "123"
2. 转为数字
使用
Number()
函数或parseInt()
/parseFloat()
1
2console.log(Number("123")); // 123
console.log(parseInt("123px")); // 123
六、字符串的迭代
1. for…of 循环
可以使用 for…of 遍历字符串的每个字符。
1
2
3for (let char of "hello") {
console.log(char); // h, e, l, l, o
}
2. 字符串拆分为数组
使用 Array.from() 或 split()
1
console.log(Array.from("hello")); // ["h", "e", "l", "l", "o"]
七、常见字符串编码与解码
1. encodeURI
和 decodeURI
编码和解码完整的 URI。
1
2let uri = "https://example.com/?name=张三";
console.log(encodeURI(uri)); // "https://example.com/?name=%E5%BC%A0%E4%B8%89"
2. encodeURIComponent
和 decodeURIComponent
编码和解码 URI 的组成部分。
1
console.log(encodeURIComponent("张三")); // "%E5%BC%A0%E4%B8%89"