string(c++)
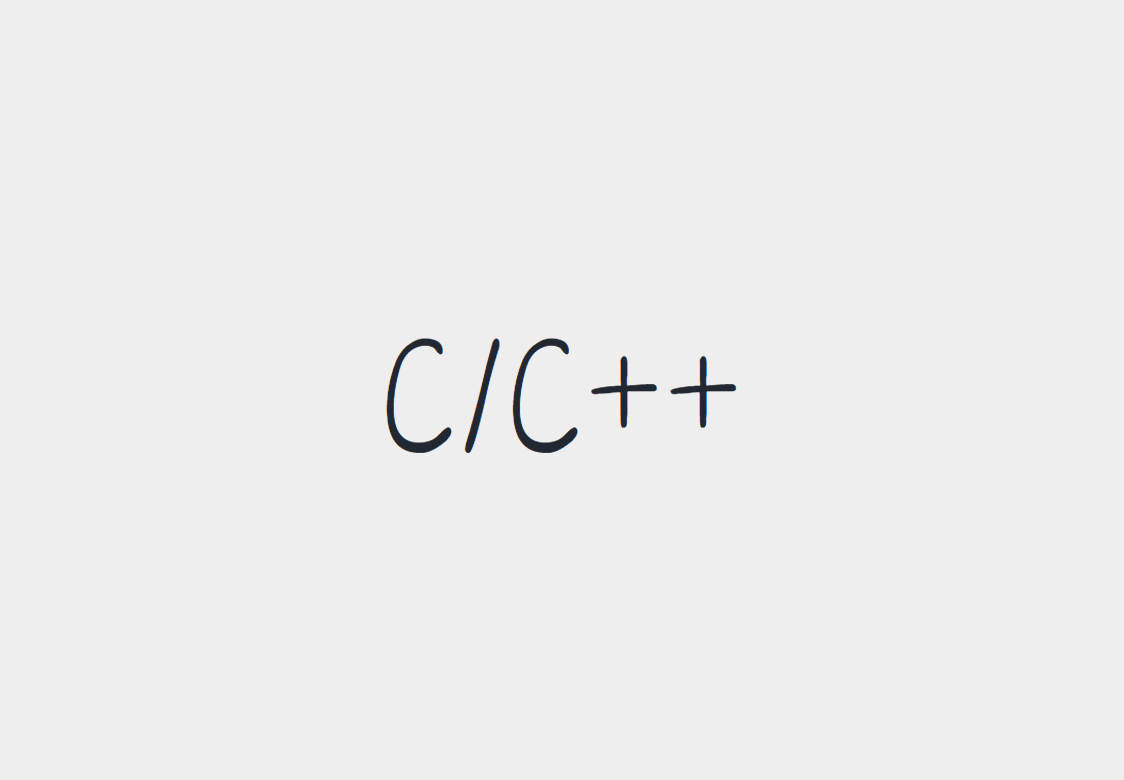
string(c++)
寻觅~流光string(c++)
一.字符串方法
1. 构造函数
string()
创建一个空字符串。string(const string& str)
复制构造函数,复制另一个字符串。string(string&& str)
移动构造函数,移动一个临时字符串。string(const char\* s)
从 C 风格字符串创建一个字符串。string(size_t n, char c)
创建一个包含n
个字符c
的字符串。
2. 赋值操作符
string& operator=(const string& str)
将另一个字符串的内容赋值给当前字符串。string& operator=(string&& str)
将另一个字符串的内容移动到当前字符串。string& operator=(const char\* s)
将 C 风格字符串s
赋值给当前字符串。
3. 访问字符
char& operator[](size_t pos)
通过下标访问字符(没有范围检查)。const char& operator[](size_t pos) const
通过下标访问字符(常量版本,不可修改)。char& at(size_t pos)
通过下标访问字符,并进行范围检查。const char& at(size_t pos) const
通过下标访问字符(常量版本,带范围检查)。
4. 修改字符串
void clear()
清空字符串内容,释放内存。void resize(size_t n)
调整字符串的大小为n
,多余部分用空字符填充。void resize(size_t n, char c)
调整字符串大小为n
,新增加的字符用c
填充。void append(const string& str)
将字符串str
追加到当前字符串。void append(const char\* s)
将 C 风格字符串s
追加到当前字符串。void append(const char\* s, size_t n)
将 C 风格字符串s
中的前n
个字符追加到当前字符串。void push_back(char c)
将字符c
追加到字符串末尾。string& operator+=(const string& str)
将字符串str
追加到当前字符串。string& operator+=(const char\* s)
将 C 风格字符串s
追加到当前字符串。
5. 查找字符串
size_t find(const string& str, size_t pos = 0) const
查找字符串str
在当前字符串中的首次出现位置。size_t find(const char\* s, size_t pos = 0) const
查找 C 风格字符串s
在当前字符串中的首次出现位置。size_t find(char c, size_t pos = 0) const
查找字符c
在当前字符串中的首次出现位置。size_t rfind(const string& str, size_t pos = string::npos) const
从字符串的末尾开始查找str
。size_t rfind(const char\* s, size_t pos = string::npos) const
从字符串的末尾开始查找 C 风格字符串s
。size_t rfind(char c, size_t pos = string::npos) const
从字符串的末尾开始查找字符c
。
6. 子字符串操作
string substr(size_t pos = 0, size_t len = string::npos) const
返回从pos
位置开始,长度为len
的子字符串。string& replace(size_t pos, size_t len, const string& str)
从pos
位置开始,替换len
长度的内容为字符串str
。string& replace(size_t pos, size_t len, const char\* s)
从pos
位置开始,替换len
长度的内容为 C 风格字符串s
。
7. 比较字符串
int compare(const string& str) const
比较当前字符串与str
,返回值:- 小于 0:当前字符串小于
str
。 - 0:当前字符串等于
str
。 - 大于 0:当前字符串大于
str
。
- 小于 0:当前字符串小于
int compare(size_t pos, size_t len, const string& str) const
比较当前字符串从pos
开始,长度为len
的部分与str
。
8. 获取字符串信息
size_t size() const
返回字符串的长度(字符数)。size_t length() const
返回字符串的长度,等同于size()
。size_t capacity() const
返回字符串当前分配的内存大小(以字符数为单位)。bool empty() const
判断字符串是否为空。const char\* c_str() const
返回一个 C 风格字符串(以 null 结尾的字符数组)。
9. 其他方法
string& erase(size_t pos = 0, size_t len = string::npos)
删除从pos
位置开始,长度为len
的部分。string& insert(size_t pos, const string& str)
在pos
位置插入字符串str
。string& insert(size_t pos, const char\* s)
在pos
位置插入 C 风格字符串s
。string& insert(size_t pos, size_t len, char c)
在pos
位置插入len
个字符c
。
10. 转换操作
string& tolower()
将字符串中的所有字母转换为小写(需手动实现,C++ 标准库没有内建方法)。string& toupper()
将字符串中的所有字母转换为大写(需手动实现,C++ 标准库没有内建方法)。
二.程序举列
1. 构造函数与赋值
1 |
|
2. 字符串修改与追加
1 |
|
3. 字符访问
1 |
|
4. 查找字符串
1 |
|
5. 子字符串
1 |
|
6. 字符串替换
1 |
|
7. 字符串比较
1 |
|
8. 字符串清空与检查是否为空
1 |
|
9. 字符串长度与容量
1 |
|
评论
匿名评论隐私政策
TwikooWaline
✅ 你无需删除空行,直接评论以获取最佳展示效果